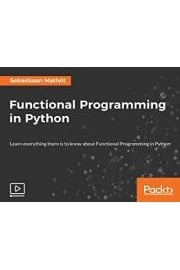
Watch Functional Programming in Python
- 2017
- 1 Season
Functional Programming in Python, from Packt Publishing, is a comprehensive guide to functional programming in Python. This show aims to teach developers the core concepts of functional programming, how they can be applied in Python, and how to use them effectively to write clean, readable, and maintainable code.
The show covers all the key concepts of functional programming: immutability, higher-order functions, pure functions, recursion, and more. It then goes on to show how to apply these concepts in the context of Python. This includes using Python's built-in functions, working with lambda functions and closures, and using Python's functional programming libraries such as functools and itertools.
Throughout the show, viewers are introduced to a variety of functional programming techniques and best practices, and given the opportunity to practice these techniques through hands-on coding exercises. The show also covers some of the common pitfalls of functional programming and how to avoid them.
The first part of the show covers the basics of functional programming, starting with an introduction to what functional programming is and its benefits. Viewers are then introduced to the core concepts of functional programming and shown how they can be applied in Python. This includes topics such as higher-order functions, recursion, and map, filter, and reduce.
The show then moves on to cover some more advanced topics, such as using closures and decorators in Python, working with generators, and handling errors and exceptions in functional programming.
One of the key benefits of functional programming is its ability to make code more modular and easier to read and maintain. The show explores this in detail, showing viewers how to write clean, concise, and reusable code using functional programming techniques. It covers topics such as abstraction, data hiding, and functional composition.
The show also covers some of the most commonly used libraries and frameworks for functional programming in Python. This includes libraries such as functools and itertools, which provide useful tools for functional programming, and frameworks such as Flask, which make it easier to build functional programming applications in Python.
One of the unique features of the show is its focus on real-world applications of functional programming in Python. Throughout the show, viewers are shown how functional programming can be used to solve real-world problems and build real-world applications. This includes topics such as data analysis, web development, and system administration.
Overall, Functional Programming in Python, from Packt Publishing, is a comprehensive and practical guide to functional programming in Python. Whether you are a beginner or an experienced Python developer, this show provides a valuable resource for learning how to write clean, readable, and maintainable code using functional programming techniques.
Functional Programming in Python is a series that is currently running and has 1 seasons (44 episodes). The series first aired on July 28, 2017.