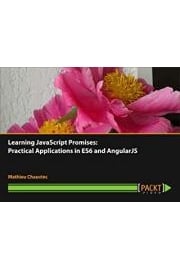
Watch Learning JavaScript Promises: Practical Applications in ES6 and AngularJS
- 2015
- 1 Season
Learning JavaScript Promises: Practical Applications in ES6 and AngularJS from Packt Publishing is a comprehensive video course that is designed to teach you how to use JavaScript Promises in practical applications. In this course, Mathieu Chauvinc, an experienced software developer and educator, will walk you through the fundamentals of JavaScript Promises and how they can be used to effectively manage asynchronous operations in your code.
The course is structured into six chapters, starting with an introduction to Promises and how they differ from traditional callbacks. You will learn about the different states of a Promise and how to handle errors and exceptions using .catch(). Mathieu will also teach you how to work with Promises in parallel and in sequence, and demonstrate how to use Promise.all() and Promise.race() to handle multiple asynchronous operations.
In chapter two, Mathieu will teach you about Promise chaining and how it can help to simplify your code. You will learn how to create chains of Promises, and how to pass data between them. Mathieu will also demonstrate how to use Promise.finally() to create clean-up functions that run regardless of whether a Promise chain resolves or rejects.
In chapter three, you will learn about Promises in ES6, including the new Promise constructor, arrow functions, and destructuring. Mathieu will also show you how to use Promise.resolve() and Promise.reject() to create Promises from existing values.
Chapter four focuses on Promises in AngularJS, an open-source front-end web application framework. You will learn how to use the $q service to create and manage Promises in AngularJS, and how to use $http to make HTTP requests that return Promises. Mathieu will also cover AngularJS promises in action, including how to use them with services and directives.
Chapter five is all about advanced Promise techniques. Mathieu will teach you about Promise composition, including how to create custom Promise combinators. He will also cover Promises with generators, and how to use them to create asynchronous iterators.
Finally, in chapter six, you will learn about Promises in production, including how to debug Promise code, how to handle Promise dependencies, and how to test Promise code using Jasmine. Mathieu will also demonstrate how to use Promises with Node.js and how to handle Promise rejections in TypeScript.
Overall, Learning JavaScript Promises: Practical Applications in ES6 and AngularJS from Packt Publishing is an in-depth course that is ideal for anyone who wants to learn how to effectively use Promises in their JavaScript projects. Whether you're a seasoned developer or new to JavaScript, this course will provide you with practical skills that you can apply to your own code.
Learning JavaScript Promises: Practical Applications in ES6 and AngularJS is a series that is currently running and has 1 seasons (20 episodes). The series first aired on October 29, 2015.